京东搜索 - 业务编写
大约 1 分钟数据库技术ElasticSearch
ContentService
public interface ContentService {
/**
* 解析数据放入ES库中
* @param keywords
* @throws IOException
* @return
*/
Boolean parseContent(String keywords) throws IOException;
/**
* 获取数据实现搜索
* @param keywords
* @param pageNo
* @param pageSize
* @return
*/
List<Map<String, Object>> searchPage(String keywords, int pageNo, int pageSize) throws IOException;
}
ContentServiceImpl
@Service
public class ContentServiceImpl implements ContentService{
@Autowired
private RestHighLevelClient restHighLevelClient;
@Autowired
private ObjectMapper objectMapper;
@Override
public Boolean parseContent(String keywords) throws IOException {
List<Content> contents = new HtmlParseUtils().parseJd(keywords);
// 数据放入es
BulkRequest bulkRequest = new BulkRequest();
bulkRequest.timeout("2m");
for (Content content : contents) {
bulkRequest.add(new IndexRequest("jd_goods")
.source(objectMapper.writeValueAsString(content), XContentType.JSON));
}
BulkResponse bulk = restHighLevelClient.bulk(bulkRequest, RequestOptions.DEFAULT);
return !bulk.hasFailures();
}
@Override
public List<Map<String, Object>> searchPage(String keywords, int pageNo, int pageSize) throws IOException {
if (pageNo <= 1){
pageNo = 1;
}
// 条件搜索
SearchRequest searchRequest = new SearchRequest("jd_goods");
SearchSourceBuilder sourceBuilder = new SearchSourceBuilder();
// 分页
sourceBuilder.from(pageNo).size(pageSize);
// 精准匹配
MatchQueryBuilder matchQueryBuilder = QueryBuilders.matchQuery("title", keywords);
sourceBuilder.query(matchQueryBuilder);
sourceBuilder.timeout(TimeValue.timeValueSeconds(5));
// 执行搜索
searchRequest.source(sourceBuilder);
SearchResponse response = restHighLevelClient.search(searchRequest, RequestOptions.DEFAULT);
// 解析结果
List<Map<String, Object>> list = new ArrayList<>();
Arrays.stream(response.getHits().getHits()).forEach((a -> list.add(a.getSourceAsMap())));
return list;
}
}
ContentController
@RestController
public class ContentController {
@Autowired
private RestHighLevelClient restHighLevelClient;
@Autowired
private ContentService contentService;
@GetMapping("/parse/{keywords}")
public boolean parse(@PathVariable("keywords") String keywords) throws IOException {
return contentService.parseContent(keywords);
}
@GetMapping("/search/{keywords}/{pageNo}/{pageSize}")
public List<Map<String, Object>> search(@PathVariable("keywords") String keywords,
@PathVariable("pageNo") int pageNo,
@PathVariable("pageSize") int pageSize) throws IOException {
return contentService.searchPage(keywords, pageNo, pageSize);
}
}
测试插入数据
http://localhost:9090/parse/java
http://localhost:9090/parse/算法
http://localhost:9090/parse/vue
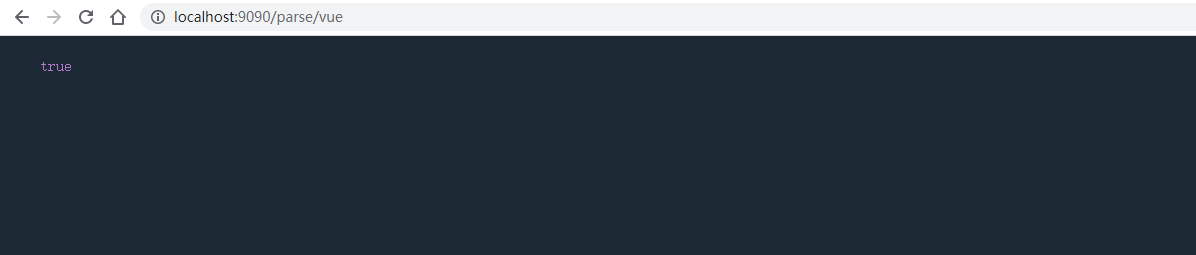
查看head页面:
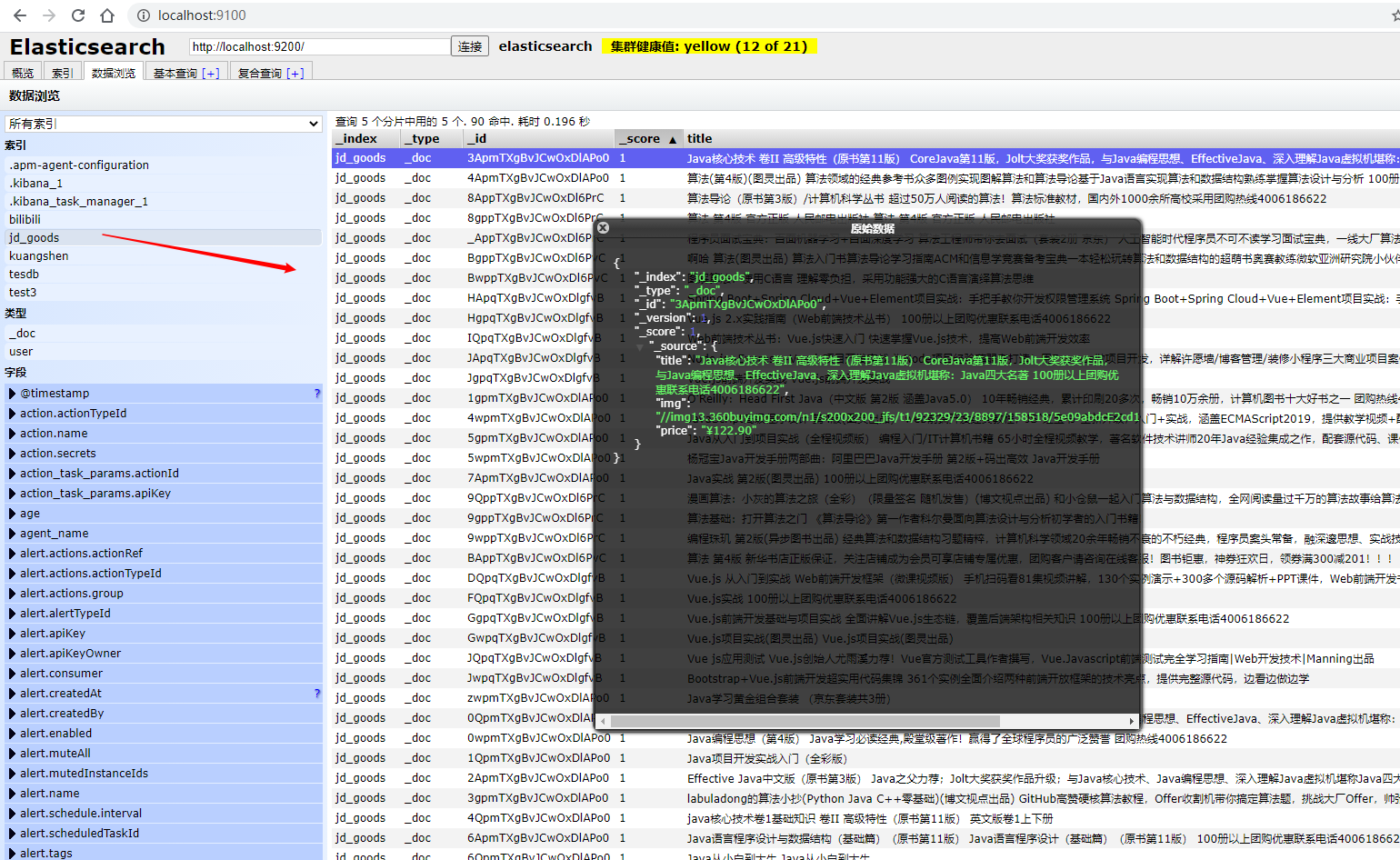
测试查询ES中的数据
http://localhost:9090/search/java/1/10
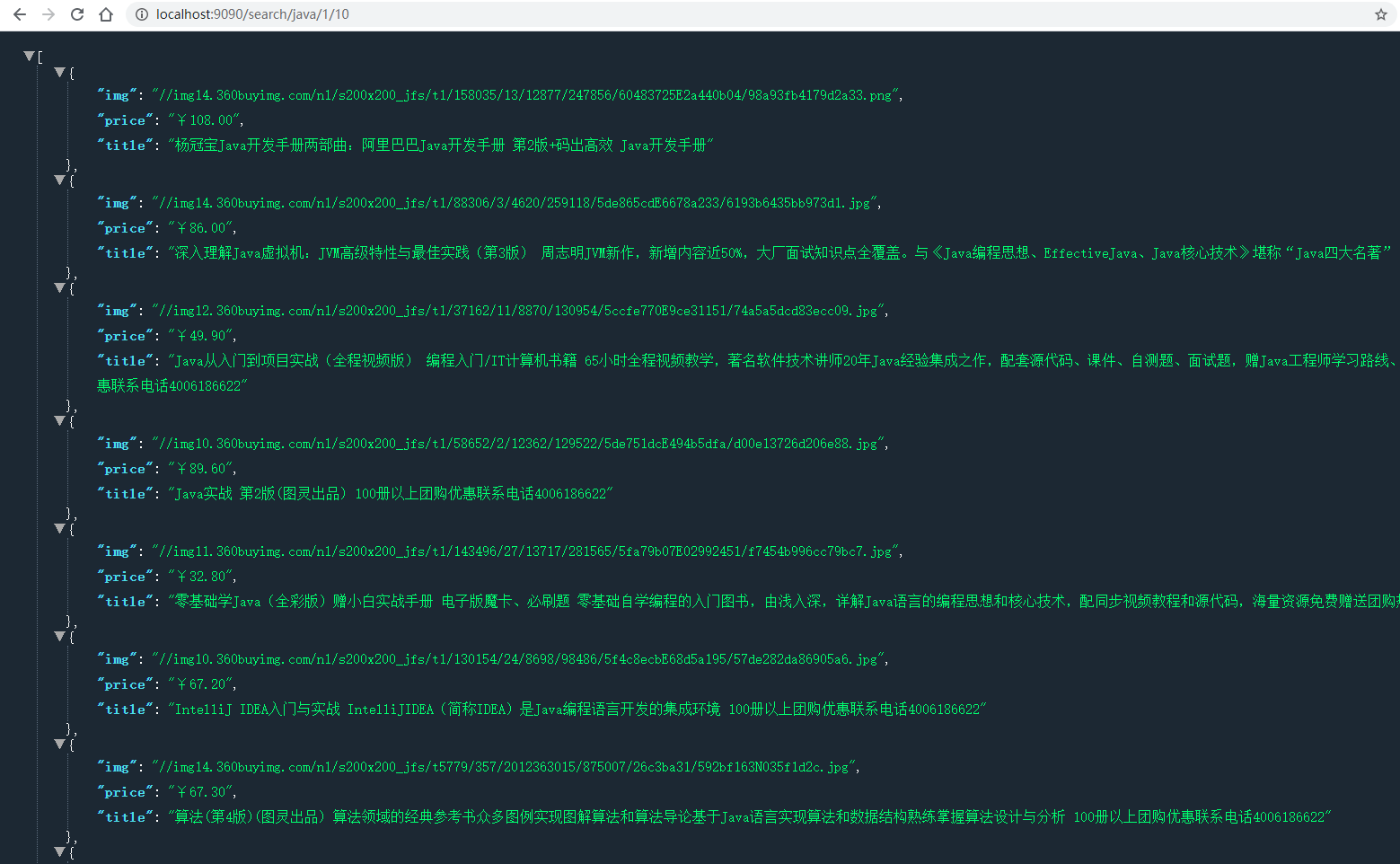
http://localhost:9090/search/算法/1/10
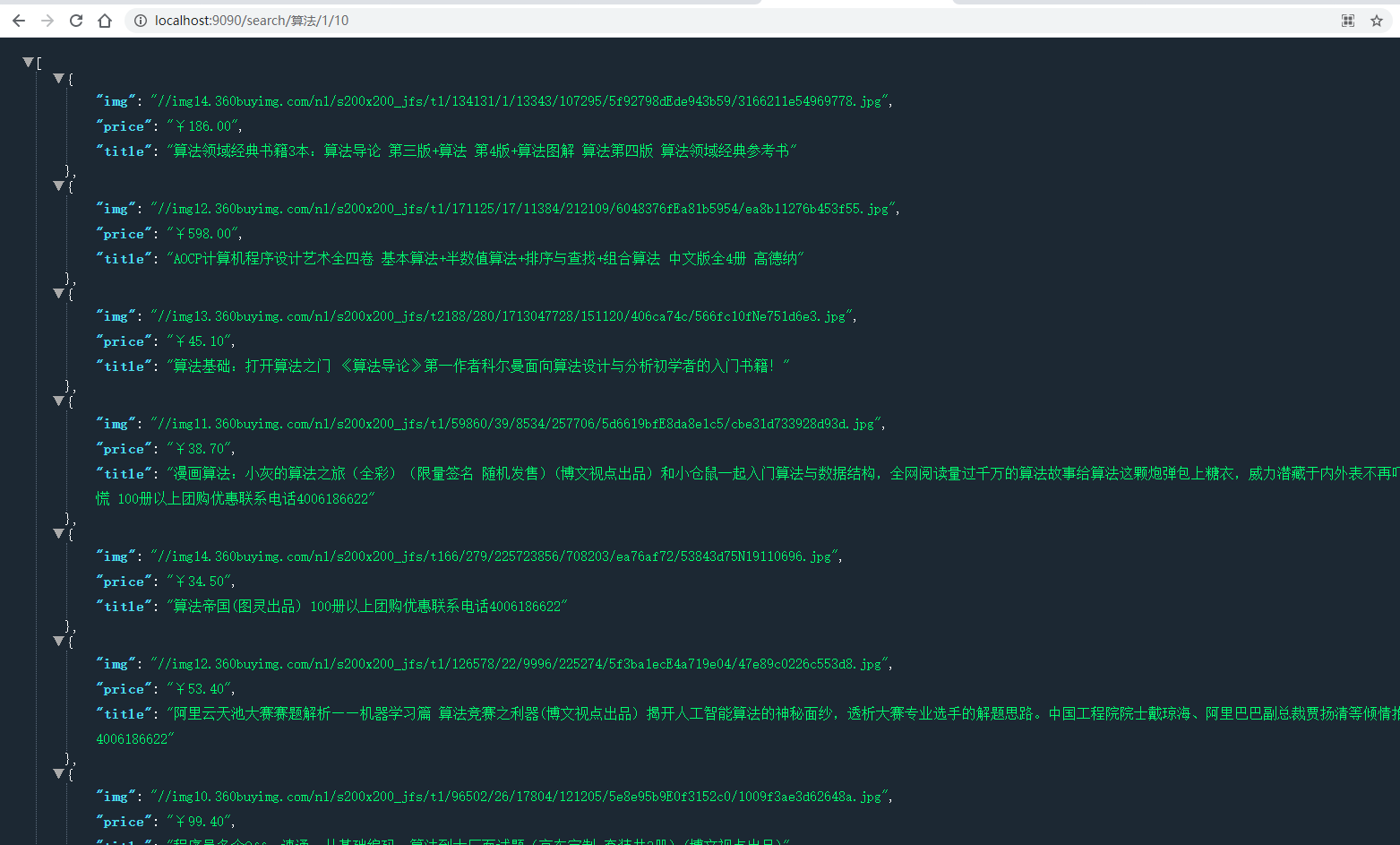