SpringBoot集成Redis
大约 2 分钟数据库技术Redis
SpringBoot操作数据:spring-data- jpa jdbc mongodb rdis
SpringData也是和SpringBoot齐名的项目。
在SpringBoot 2.x之后,原来使用的Jedis被替换成了
lettuce
。
- Jedis底层是采用的直连,多个线程操作的话,是不安全的。如果想要避免不安全,使用Jedis pool连接池。更像BIO模式。
lettuce采用netty,实例可以在多个线程中进行共享。不存在线程不安全的情况。可以减少线程数量。更像NIO模式。
源码分析
@Configuration(proxyBeanMethods = false)
@ConditionalOnClass(RedisOperations.class)
@EnableConfigurationProperties(RedisProperties.class)
@Import({ LettuceConnectionConfiguration.class, JedisConnectionConfiguration.class })
public class RedisAutoConfiguration {
@Bean
@ConditionalOnMissingBean(name = "redisTemplate") // 可以自己定义RedisTemplate替换默认的
@ConditionalOnSingleCandidate(RedisConnectionFactory.class)
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
// 默认的RedisTemplate没有过多的设置.redis对象都是需要序列化。
// 两个泛型都是Object, Object,之后使用需要强制转换
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
@Bean
@ConditionalOnMissingBean
@ConditionalOnSingleCandidate(RedisConnectionFactory.class)
// 由于String是redis中最常使用的类型,所以单独提出来了一个Bean
public StringRedisTemplate stringRedisTemplate(RedisConnectionFactory redisConnectionFactory) {
StringRedisTemplate template = new StringRedisTemplate();
template.setConnectionFactory(redisConnectionFactory);
return template;
}
}
整合测试
1、导入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
2、配置连接
spring.redis.host=127.0.0.1
spring.redis.port=6379
3、测试
@SpringBootTest
class Redis02SpringbootApplicationTests {
@Autowired
private RedisTemplate redisTemplate;
@Test
void contextLoads() {
/**
* opsForValue 操作String
* opsForList 操作List
* opsForSet
* opsForHash
* opsForZSet
* opsForGeo
* opsForHyperLogLog
* 获取连接对象
*/
RedisConnection connection = redisTemplate.getConnectionFactory().getConnection();
System.out.println(connection.ping());
redisTemplate.opsForValue().set("mykey", "哔哩哔哩");
System.out.println(redisTemplate.opsForValue().get("mykey"));
}
}
结果
PONG
哔哩哔哩
2020-12-30 11:00:52.653 INFO 16896 --- [extShutdownHook] o.s.s.concurrent.ThreadPoolTaskExecutor : Shutting down ExecutorService 'applicationTaskExecutor'
Process finished with exit code 0
序列化配置
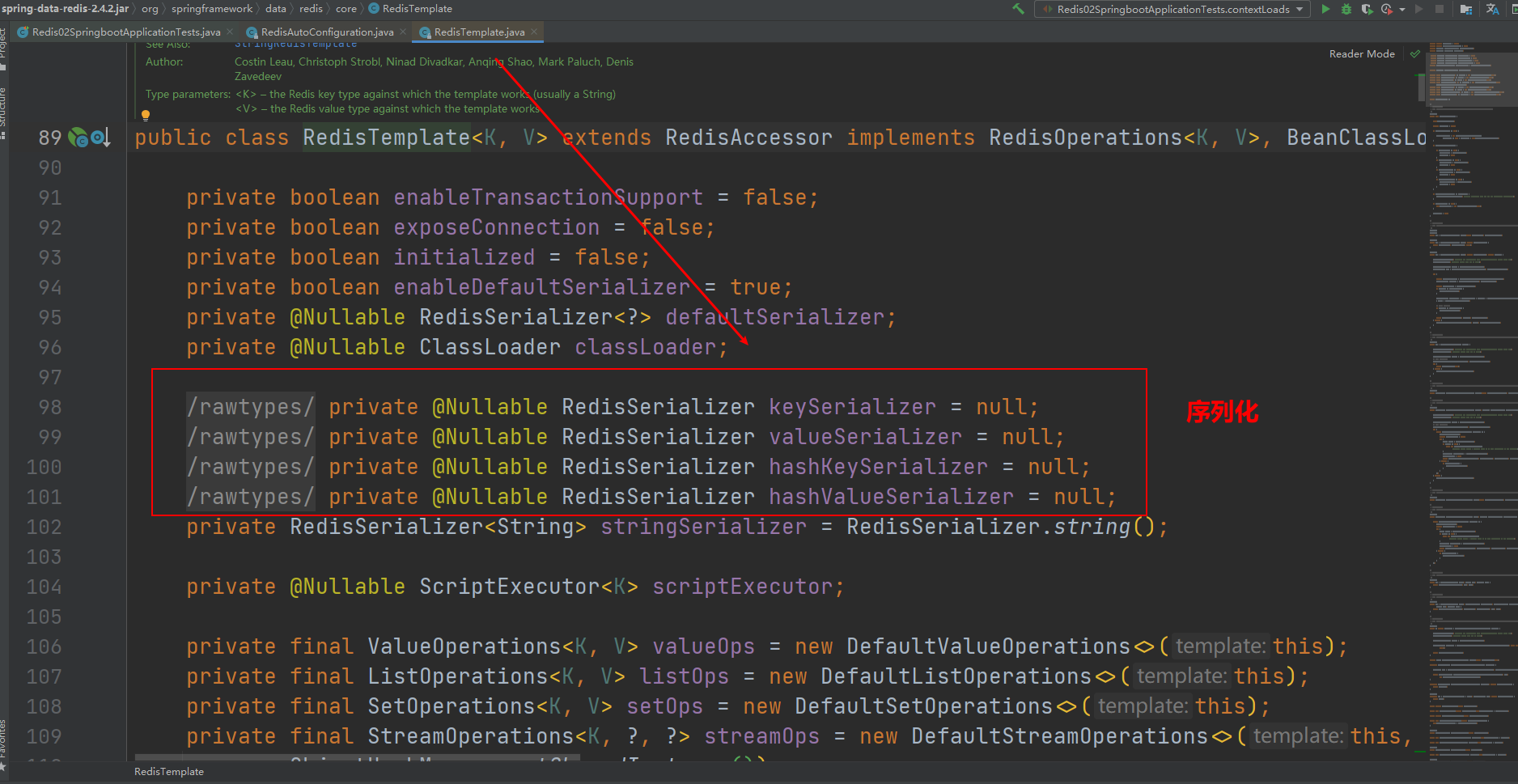
默认的序列化方式
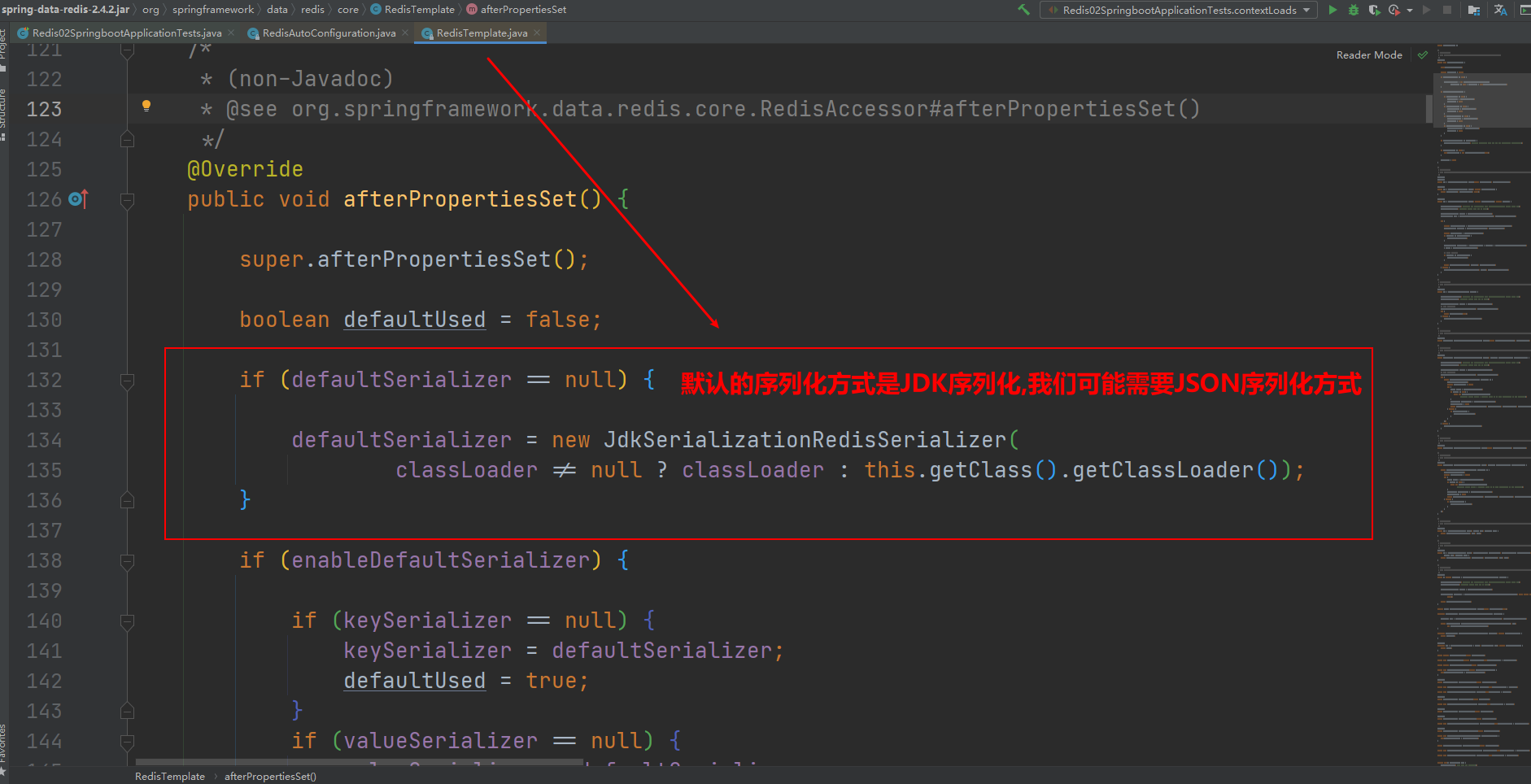