整合Redis
大约 2 分钟Spring全家桶SpringBoot精讲细讲
引入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
配置文件
application.yaml
spring:
redis:
host: 127.0.0.1
StringRedisTemplate使用
@SpringBootTest
class SpringbootIntegrationFrameworkApplicationTests {
@Autowired
private StringRedisTemplate stringRedisTemplate;
@Test
void contextLoads() {
BoundValueOperations<String, String> user = stringRedisTemplate.boundValueOps("user");
user.set("1");
user.increment();
String s = user.get();
System.out.println(s);
user.decrement();
user.setIfAbsent("123");
user.setIfPresent("222333");
System.out.println(user.get());
}
}
结果
2
222333
查看redis(使用的是Windows的RDM软件):
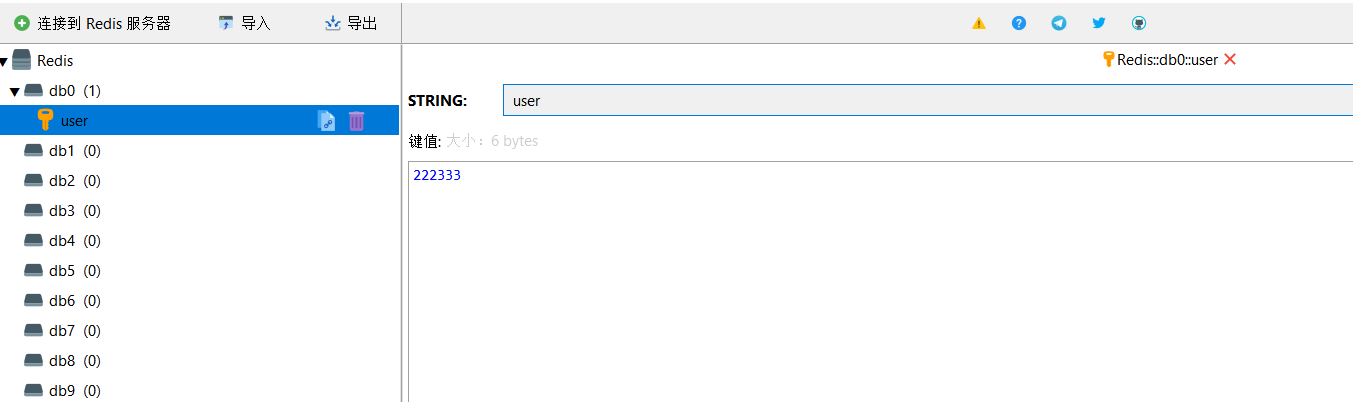
整合自定义RedisTemplate
使用StringRedisTemplate
变成String是一种序列化。
使用jackson先把对象转化成JSON字符串。然后把字符串存到redis中。读取redis中的数据时,读到的是字符串,使用工具转成对象。
@SpringBootTest
class SpringbootIntegrationFrameworkApplicationTests {
@Autowired
private StringRedisTemplate stringRedisTemplate;
@Test
void contextLoads() throws JsonProcessingException {
BoundHashOperations<String, Object, Object> users = stringRedisTemplate.boundHashOps("users");
User user = new User(10L, "赵六", "123456", "sdghg", new Date(), 10, new Date(), new Date());
ObjectMapper objectMapper = new ObjectMapper();
String userStr = objectMapper.writeValueAsString(user);
users.put("user:1", userStr);
String user1 = (String) users.get("user:1");
System.out.println(user1);
// 将从redis读取的字符串转成User对象
User user2 = objectMapper.readValue(user1, User.class);
System.out.println(user2);
}
}
结果
{"id":10,"username":"赵六","password":"123456","salt":"sdghg","birth":1620821229457,"deptId":10,"createTime":1620821229457,"updateTime":1620821229457}
User(id=10, username=赵六, password=123456, salt=sdghg, birth=Wed May 12 20:07:09 CST 2021, deptId=10, createTime=Wed May 12 20:07:09 CST 2021, updateTime=Wed May 12 20:07:09 CST 2021)
查看redis
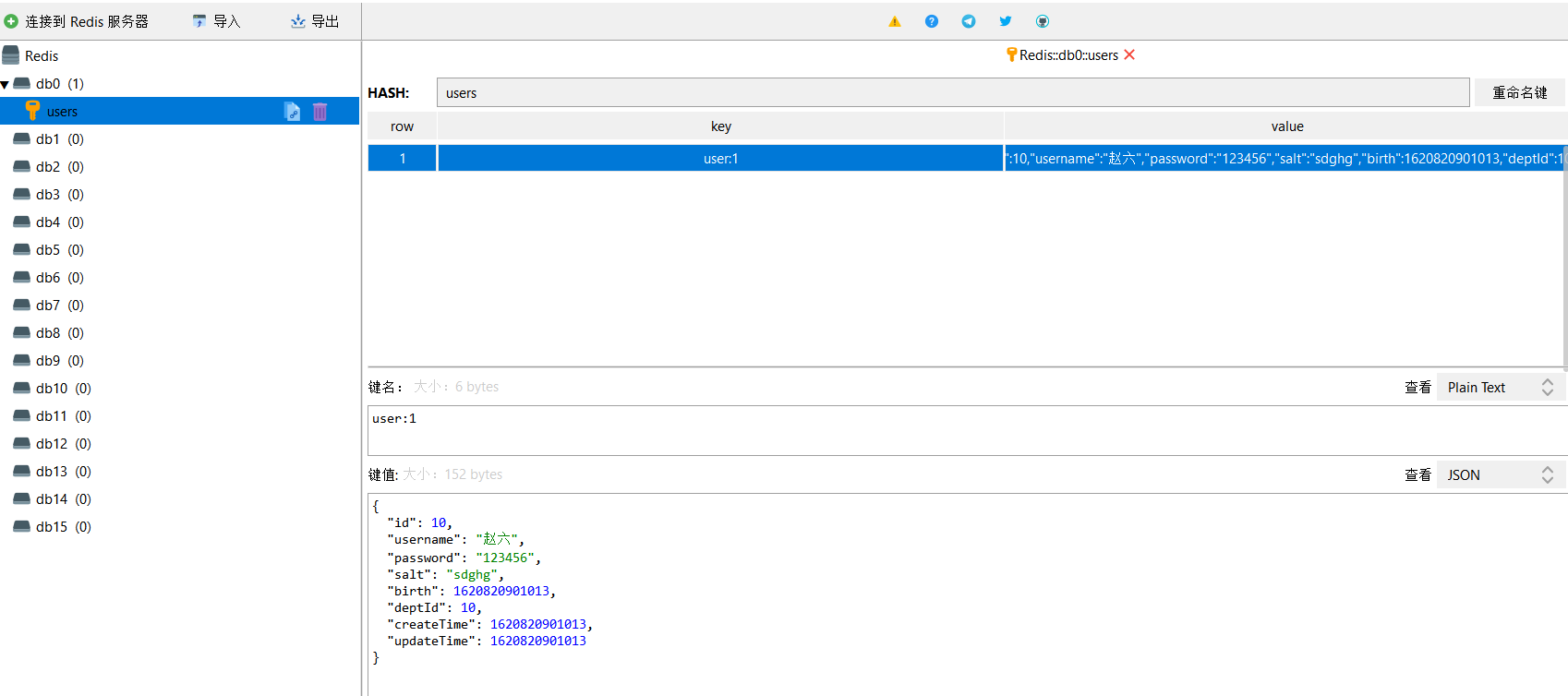
使用RedisTemplate
变成二进制是一种序列化。
@SpringBootTest
class SpringbootIntegrationFrameworkApplicationTests {
@Resource
private RedisTemplate<String, Object> redisTemplate;
@Test
void contextLoads() throws JsonProcessingException {
User user = new User(10L, "赵六", "123456", "sdghg", new Date(), 10, new Date(), new Date());
BoundValueOperations<String, Object> a = redisTemplate.boundValueOps("a");
a.set(user);
System.out.println(a.get());
}
}
结果
User(id=10, username=赵六, password=123456, salt=sdghg, birth=Wed May 12 20:23:43 CST 2021, deptId=10, createTime=Wed May 12 20:23:43 CST 2021, updateTime=Wed May 12 20:23:43 CST 2021)
查看redis

自定义RedisTemplate实现存取数据
config配置类
@Configuration
public class RedisConfig {
@Bean("MyRedisTemplate")
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory){
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(factory);
// 设置序列化的方式
redisTemplate.setKeySerializer(RedisSerializer.string());
redisTemplate.setHashKeySerializer(RedisSerializer.string());
redisTemplate.setValueSerializer(RedisSerializer.json());
redisTemplate.setHashValueSerializer(RedisSerializer.json());
return redisTemplate;
}
}
使用
@SpringBootTest
class SpringbootIntegrationFrameworkApplicationTests {
@Autowired
@Qualifier("MyRedisTemplate")
private RedisTemplate<String, Object> redisTemplate;
@Test
void contextLoads() throws JsonProcessingException {
User user = new User(10L, "赵六", "123456", "sdghg", new Date(), 10, new Date(), new Date());
BoundValueOperations<String, Object> b = redisTemplate.boundValueOps("b");
b.set(user);
System.out.println(b.get());
}
}
结果:
User(id=10, username=赵六, password=123456, salt=sdghg, birth=Wed May 12 20:36:22 CST 2021, deptId=10, createTime=Wed May 12 20:36:22 CST 2021, updateTime=Wed May 12 20:36:22 CST 2021)
查看redis:
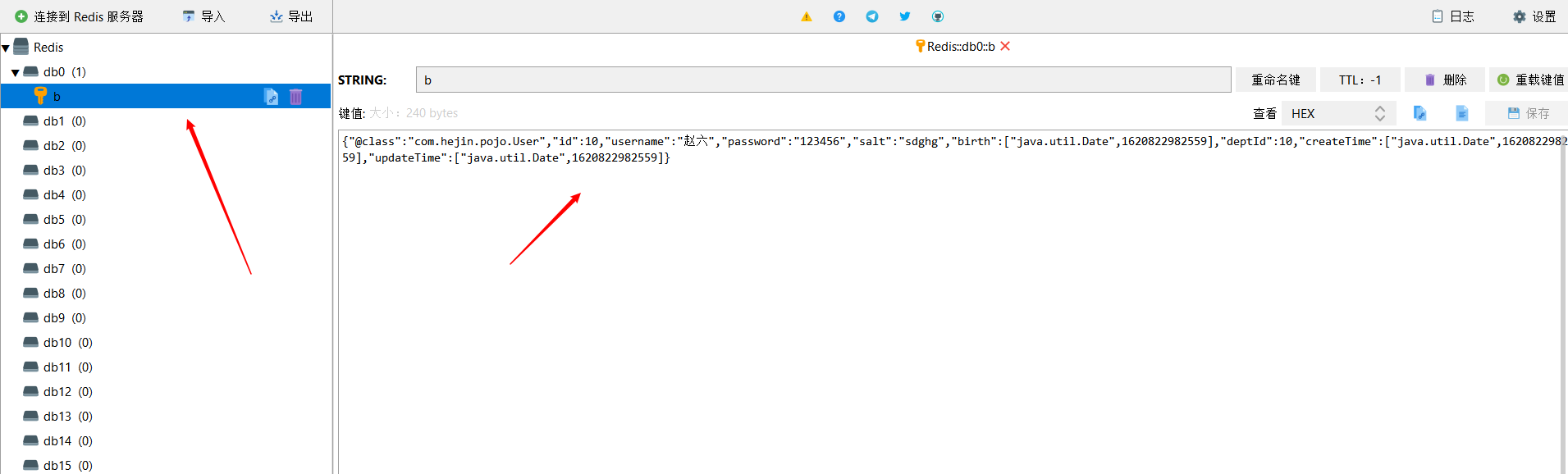