配置文件
大约 2 分钟Spring全家桶SpringBoot精讲细讲
yaml可以直接给实体类赋值。
@ConfigurationProperties IDEA爆红
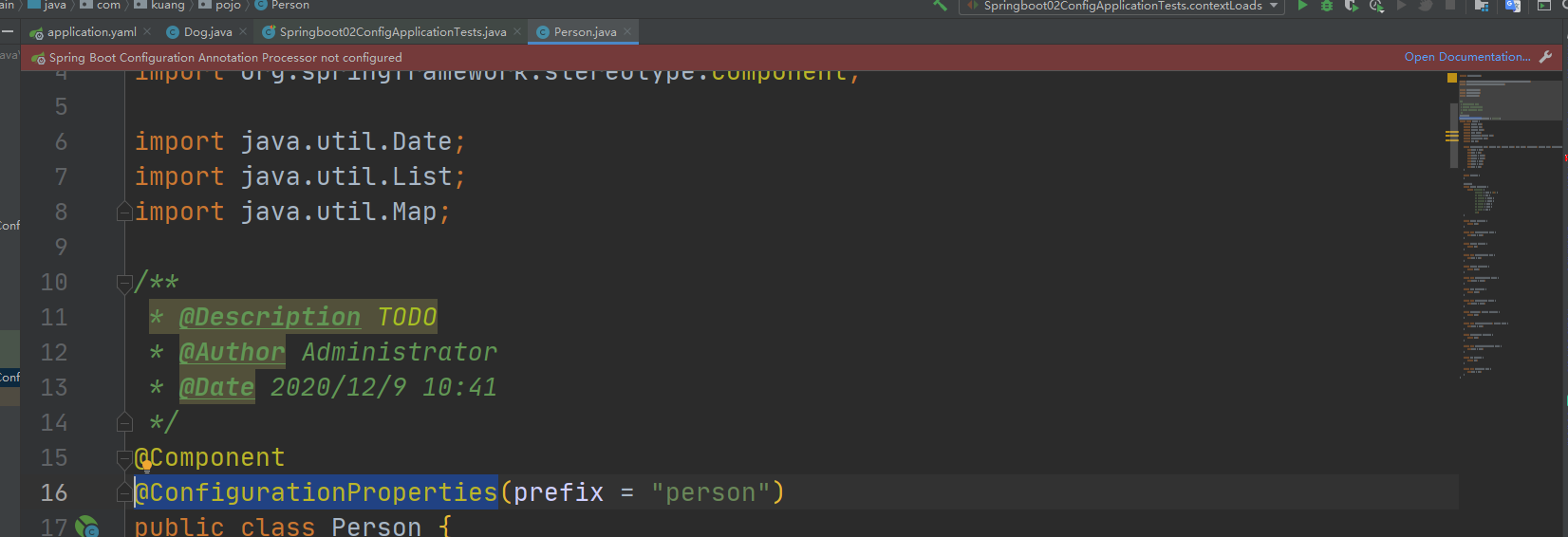
导入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
@ConfigurationProperties
绑定yaml赋值
application.yaml
person:
name: hejin${random.uuid}
age: ${random.int}
happy: false
birth: 2020/12/09
maps: {k1: v1,k2: v2,k3: v3}
list:
- code
- music
- girl
dog:
name: ${person.hello:hello}_旺旺
age: 3
Person.java
/**
* @Description TODO
* @Author Administrator
* @Date 2020/12/9 10:41
*/
@Component
@ConfigurationProperties(prefix = "person")
public class Person {
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> list;
private Dog dog;
public Person(String name, Integer age, Boolean happy, Date birth, Map<String, Object> maps, List<Object> list, Dog dog) {
this.name = name;
this.age = age;
this.happy = happy;
this.birth = birth;
this.maps = maps;
this.list = list;
this.dog = dog;
}
public Person() {
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", happy=" + happy +
", birth=" + birth +
", maps=" + maps +
", list=" + list +
", dog=" + dog +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Boolean getHappy() {
return happy;
}
public void setHappy(Boolean happy) {
this.happy = happy;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public Map<String, Object> getMaps() {
return maps;
}
public void setMaps(Map<String, Object> maps) {
this.maps = maps;
}
public List<Object> getList() {
return list;
}
public void setList(List<Object> list) {
this.list = list;
}
public Dog getDog() {
return dog;
}
public void setDog(Dog dog) {
this.dog = dog;
}
}
测试
@SpringBootTest
class Springboot02ConfigApplicationTests {
@Autowired
private Dog dog;
@Autowired
private Person person;
@Test
void contextLoads() {
System.out.println(person);
}
}
结果
Person{name='hejin24d6439b-6fa0-4aec-b3a5-b6ba2b67699e', age=-78022062, happy=false, birth=Wed Dec 09 00:00:00 CST 2020, maps={k1=v1, k2=v2, k3=v3}, list=[code, music, girl], dog=Dog{name='hello_旺旺', age=3}}
2020-12-09 11:22:12.497 INFO 11948 --- [extShutdownHook] o.s.s.concurrent.ThreadPoolTaskExecutor : Shutting down ExecutorService 'applicationTaskExecutor'
Process finished with exit code 0
@PropertySource
加载自定义properties配置文件
hejin.properties
name=哔哩哔哩
Person.java
/**
* @Description TODO
* @Author Administrator
* @Date 2020/12/9 10:41
*/
@Component
// 加载指定的配置文件
@PropertySource(value = "classpath:hejin.properties")
public class Person {
// SPEL取出配置文件的值
@Value("${name}")
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> list;
private Dog dog;
public Person(String name, Integer age, Boolean happy, Date birth, Map<String, Object> maps, List<Object> list, Dog dog) {
this.name = name;
this.age = age;
this.happy = happy;
this.birth = birth;
this.maps = maps;
this.list = list;
this.dog = dog;
}
public Person() {
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", happy=" + happy +
", birth=" + birth +
", maps=" + maps +
", list=" + list +
", dog=" + dog +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Boolean getHappy() {
return happy;
}
public void setHappy(Boolean happy) {
this.happy = happy;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public Map<String, Object> getMaps() {
return maps;
}
public void setMaps(Map<String, Object> maps) {
this.maps = maps;
}
public List<Object> getList() {
return list;
}
public void setList(List<Object> list) {
this.list = list;
}
public Dog getDog() {
return dog;
}
public void setDog(Dog dog) {
this.dog = dog;
}
}