中间操作
大约 5 分钟函数式编程stream流
filter
可以对流中的元素进行条件过滤,符合过滤条件的才能继续留在流中。
打印所有姓名长度大于1的作家的姓名:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.filter(author -> author.getName().length() > 1)
.forEach(author -> System.out.println(author.getName()));
}
结果:
蒙多
亚拉索
Process finished with exit code 0
IDEA调试结果:
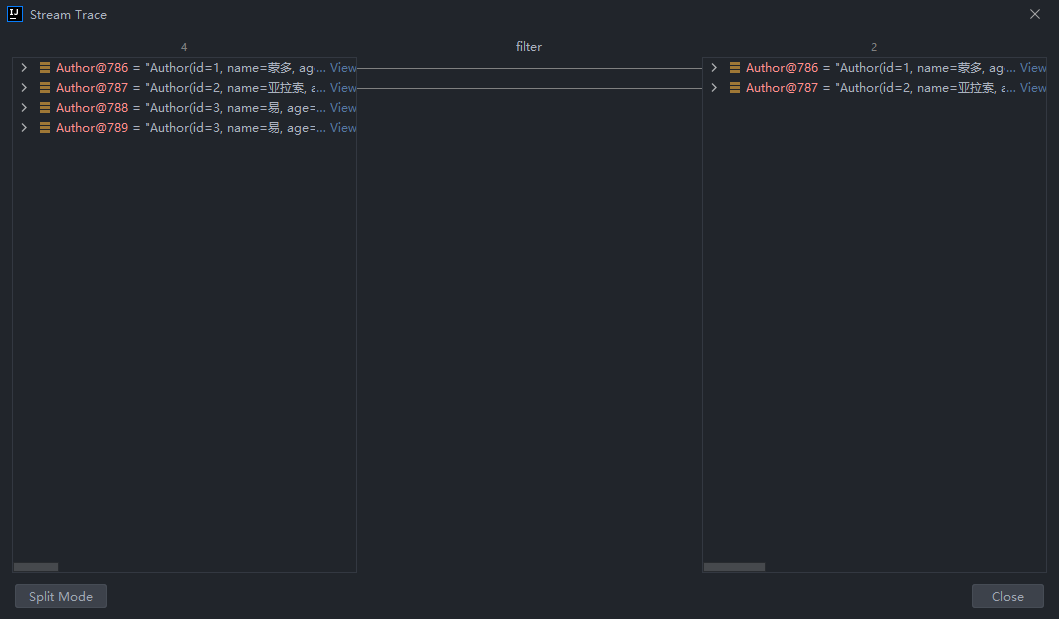
map
可以对流中的元素进行计算或者转换。
打印所有作家的姓名:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.forEach(name -> System.out.println(name));
}
结果:
蒙多
亚拉索
易
易
Process finished with exit code 0
IDEA调试:
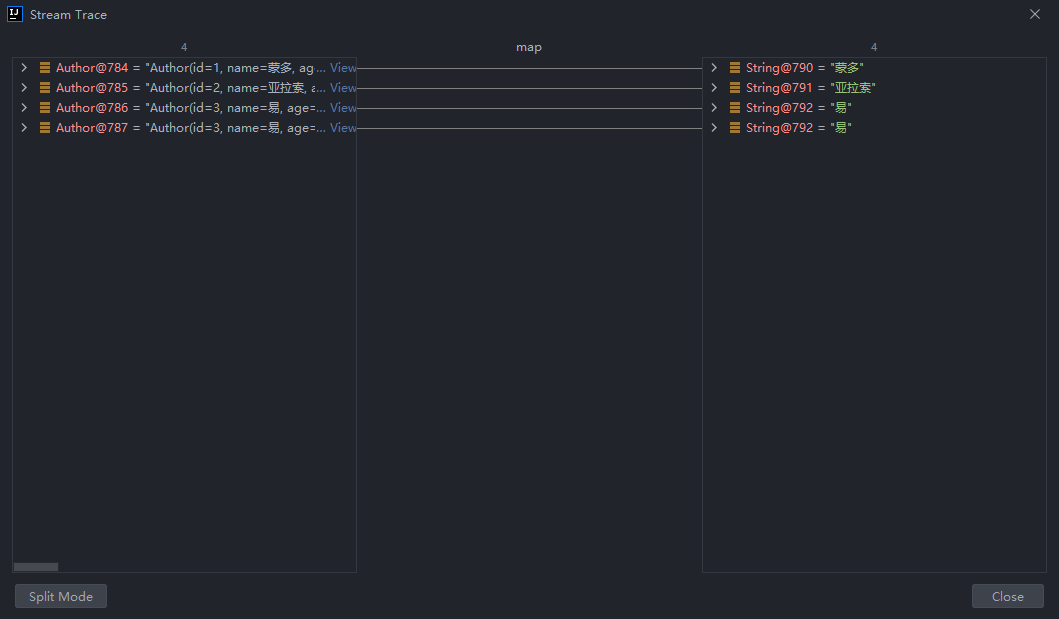
distinct
可以去除流中的重复元素。
打印作家的性别,要求不能有重复元素:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.distinct()
.forEach(name -> System.out.println(name));
}
结果:
蒙多
亚拉索
易
Process finished with exit code 0
IDEA调试结果:
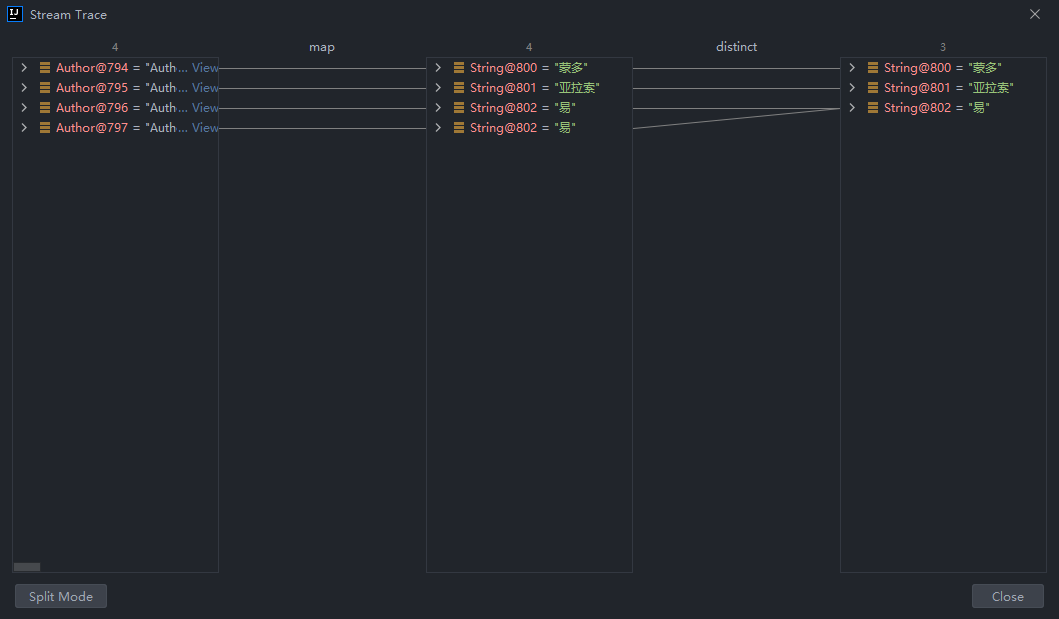
注意
distinct方法是依赖Object的equals方法来判断相同对象的,所以需要注意重写equals方法。
sorted
可以对流中的元素进行排序。
对流中的元素按照年龄进行降序排列,并且要求不能有重复的元素:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.forEach(author -> System.out.println(author));
}
结果:
Author(id=1, name=蒙多, age=33, intro=一个从菜刀中明悟哲理的祖安人, books=[Book(id=1, name=刀的两侧是光明与黑暗, category=哲学,爱情, score=88, intro=用一把刀划分了爱恨), Book(id=2, name=一个人不能死在同一把刀下, category=个人成长,爱情, score=99, intro=讲述如何从失败中明悟真理)])
Author(id=2, name=亚拉索, age=15, intro=狂风也追逐不上他的思考速度, books=[Book(id=3, name=那风吹不到的地方, category=哲学, score=85, intro=带你用思维去领略世界的尽头), Book(id=4, name=吹或不吹, category=爱情,个人传记, score=56, intro=一个哲学家的恋爱观注定很难把他所在的时代理解)])
Author(id=3, name=易, age=14, intro=是这个世界在限制他的思维, books=[Book(id=5, name=你的剑就是我的剑, category=爱情, score=56, intro=无法想象一个武者能对他的伴侣这么的宽容), Book(id=6, name=风与剑, category=个人传记, score=100, intro=两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?), Book(id=6, name=风与剑, category=个人传记, score=100, intro=两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?)])
Process finished with exit code 0
IDEA调试:
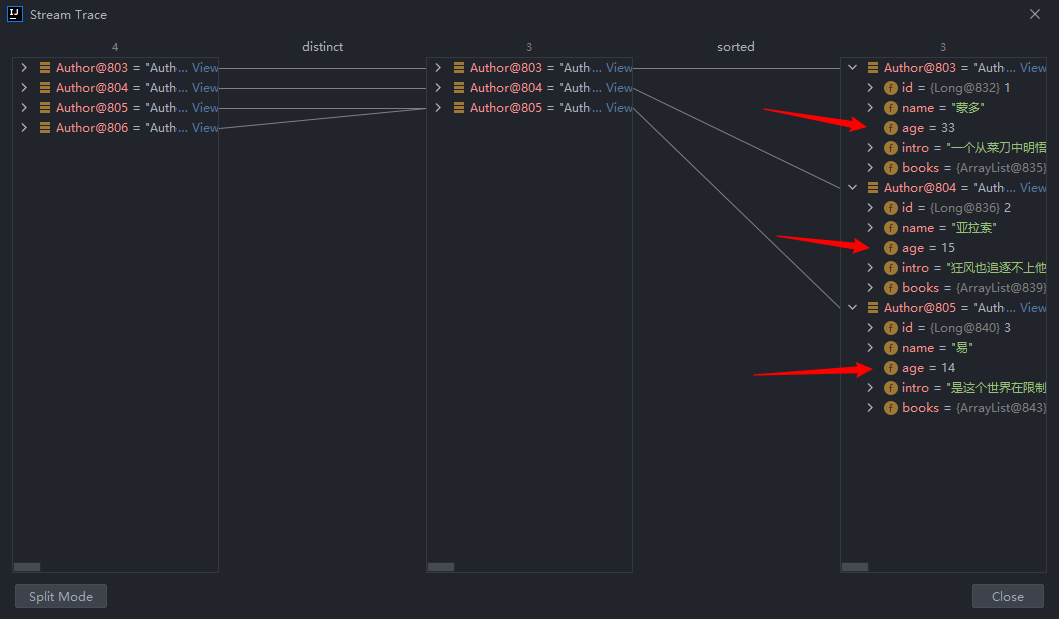
如果调用空参的sorted方法,需要流中的元素是实现了Comparable接口。
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted()
.forEach(author -> System.out.println(author));
}
Author类:
@Data
@AllArgsConstructor
@NoArgsConstructor
@EqualsAndHashCode
public class Author implements Comparable<Author>{
/**
* id
*/
private Long id;
/**
* 姓名
*/
private String name;
/**
* 年龄
*/
private int age;
/**
* 简介
*/
private String intro;
/**
* 作品
*/
private List<Book> books;
@Override
public int compareTo(Author o) {
return o.getAge() - this.getAge();
}
}
limit
可以设置流的最大长度,超出的部分将被抛弃
对流中的元素按照年龄进行降序排序,要求不能有重复的元素,打印年龄最大的2个作家的姓名:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.map(author -> author.getName())
.limit(2)
.forEach(name -> System.out.println(name));
}
结果:
蒙多
亚拉索
Process finished with exit code 0
IDEA调试:
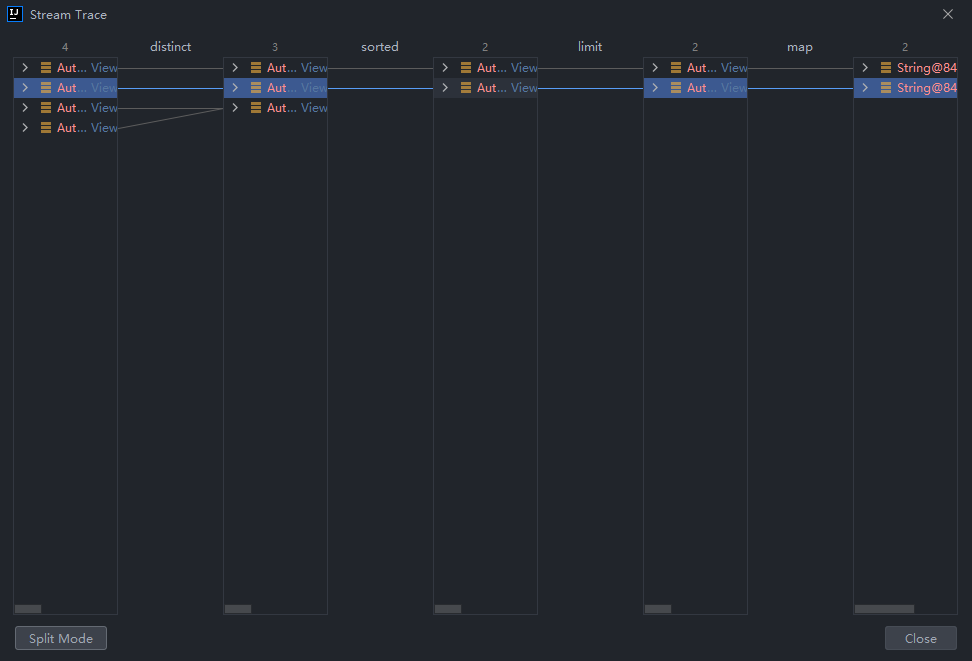
skip
跳过流中的前n的元素,返回剩下的元素。
打印出了最大年龄之外的其他作家,要求不能有重复元素,并且按照年龄降序排列:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge() - o1.getAge())
.skip(1)
.forEach(author -> System.out.println(author));
}
结果:
Author(id=2, name=亚拉索, age=15, intro=狂风也追逐不上他的思考速度, books=[Book(id=3, name=那风吹不到的地方, category=哲学, score=85, intro=带你用思维去领略世界的尽头), Book(id=4, name=吹或不吹, category=爱情,个人传记, score=56, intro=一个哲学家的恋爱观注定很难把他所在的时代理解)])
Author(id=3, name=易, age=14, intro=是这个世界在限制他的思维, books=[Book(id=5, name=你的剑就是我的剑, category=爱情, score=56, intro=无法想象一个武者能对他的伴侣这么的宽容), Book(id=6, name=风与剑, category=个人传记, score=100, intro=两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?), Book(id=6, name=风与剑, category=个人传记, score=100, intro=两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?)])
Process finished with exit code 0
IDEA调试:
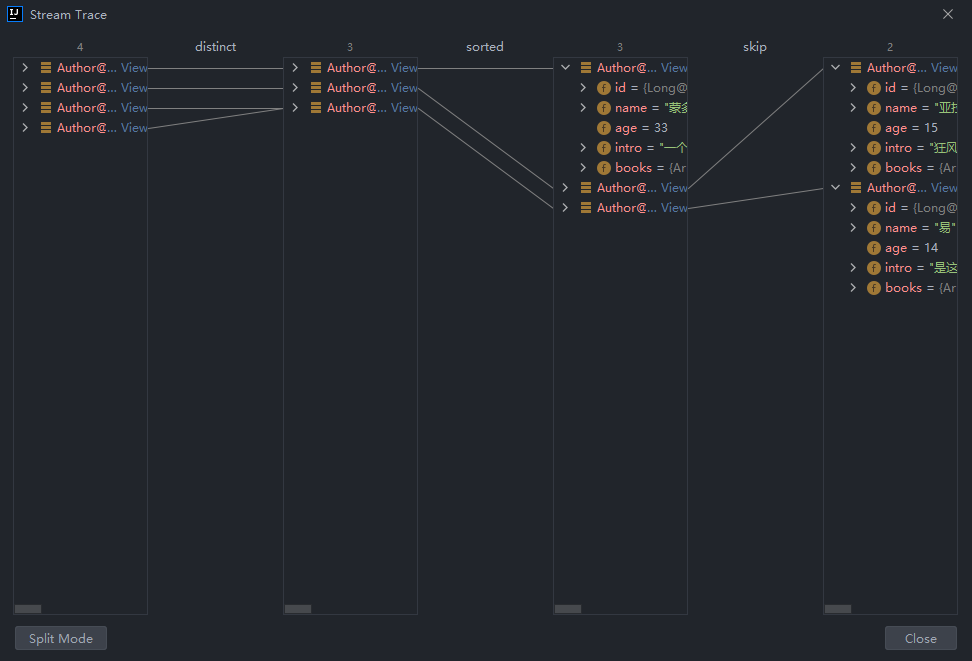
flatMap
map只能把一个对象转换成另一个对象来作为流中的元素,而flatMap可以把一个对象转换成多个对象作为流中的元素。
打印所有书籍的名字,要求对重复的元素去重:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.forEach(book -> System.out.println(book.getName()));
}
结果:
刀的两侧是光明与黑暗
一个人不能死在同一把刀下
那风吹不到的地方
吹或不吹
你的剑就是我的剑
风与剑
Process finished with exit code 0
IDEA调试:
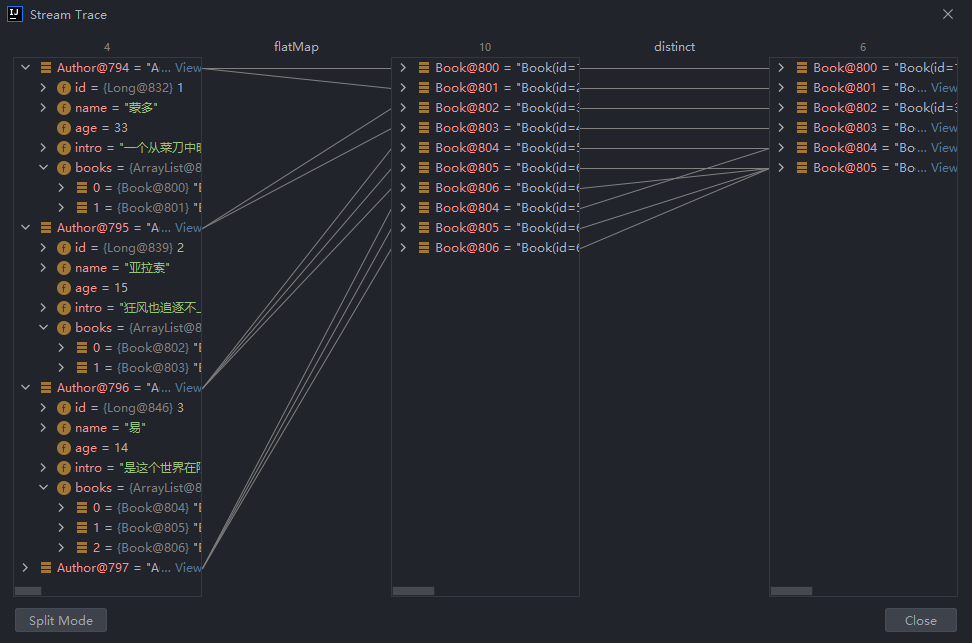
打印现有书籍的所有分类,要求对分类进行去重,不能出现这种格式:哲学,爱情:
public static void main(String[] args) {
List<Author> authors = getAuthors();
authors.stream()
.flatMap( author -> author.getBooks().stream())
.distinct()
.map(book -> book.getCategory().split(","))
.flatMap(category -> Arrays.stream(category))
.distinct()
.forEach(category -> System.out.println(category));
}
结果:
哲学
爱情
个人成长
个人传记
Process finished with exit code 0
IDEA调试:
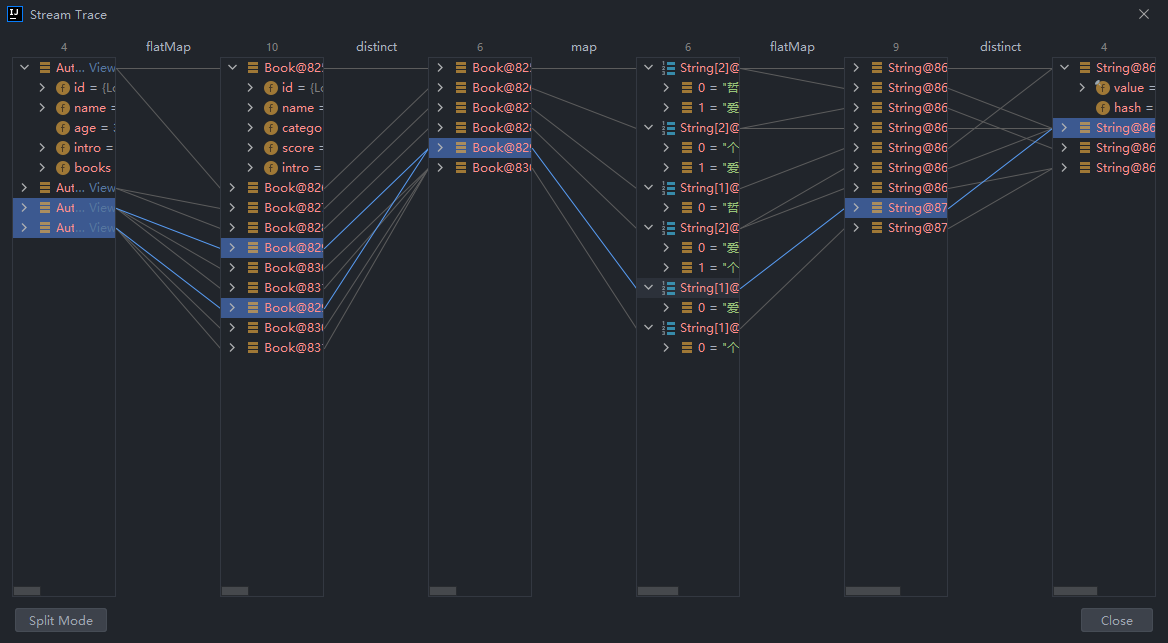