异步回调
大约 1 分钟JavaJUC并发编程
Future设计的初衷:对将来的某个事件的结果进行建模。
Future接口实现类CompletableFuture
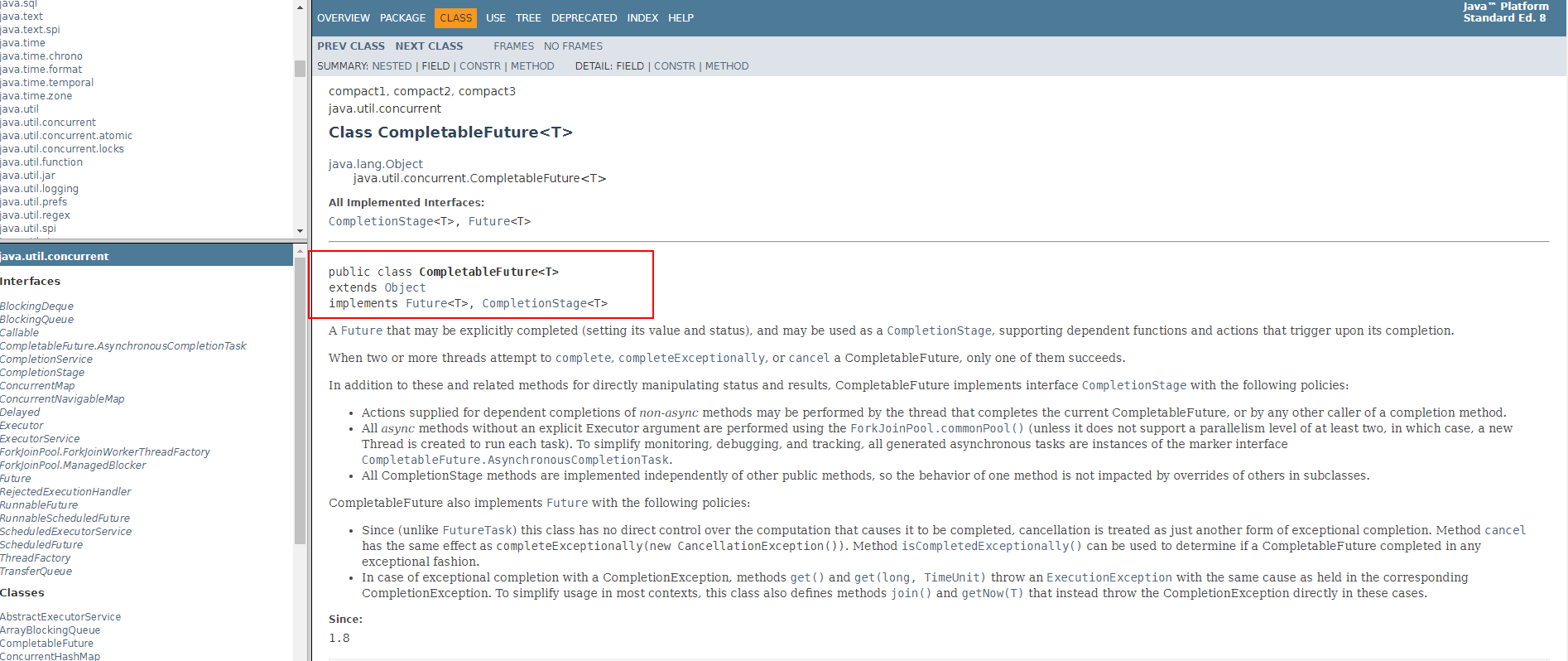
没有返回值的异步回调
public class Demo01 {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// 没有返回值的异步回调
CompletableFuture<Void> completableFuture = CompletableFuture.runAsync(() -> {
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+" runAsync==>void");
});
System.out.println("123456");
// 阻塞,获取执行结果
completableFuture.get();
}
}
结果:
123455
ForkJoinPool.commonPool-worker-1 runAsync==>void
Process finished with exit code 0
有返回值的异步回调
正常执行
public class Demo01 {
public static void main(String[] args) throws ExecutionException, InterruptedException {
/**
* 有返回值的异步回调
* ajax 成功或者失败的回调
*/
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println(Thread.currentThread().getName()+" supplyAsync==>Integer");
return 1024;
});
Integer rst = completableFuture.whenComplete((t, u) -> {
// 正常的返回结果
System.out.println("t:" + t);
System.out.println("u:" + u);
}).exceptionally((e) -> {
System.out.println(e.getMessage());
return 233;
}).get();
System.out.println(rst);
}
}
结果:
ForkJoinPool.commonPool-worker-1 supplyAsync==>Integer
t:1024
u:null
1024
Process finished with exit code 0
执行有异常
public class Demo01 {
public static void main(String[] args) throws ExecutionException, InterruptedException {
/**
* 有返回值的异步回调
* ajax 成功或者失败的回调
*/
CompletableFuture<Integer> completableFuture = CompletableFuture.supplyAsync(() -> {
System.out.println(Thread.currentThread().getName()+" supplyAsync==>Integer");
int i = 10 / 0;
return 1024;
});
Integer rst = completableFuture.whenComplete((t, u) -> {
// 正常的返回结果
System.out.println("t:" + t);
// 错误信息
System.out.println("u:" + u);
}).exceptionally((e) -> {
System.out.println(e.getMessage());
// 获取到错误的返回结果
return 233;
}).get();
System.out.println(rst);
}
}
结果:
ForkJoinPool.commonPool-worker-1 supplyAsync==>Integer
t:null
u:java.util.concurrent.CompletionException: java.lang.ArithmeticException: / by zero
java.lang.ArithmeticException: / by zero
233
Process finished with exit code 0