四大函数式接口
大约 2 分钟JavaJUC并发编程
新时代的程序员:lambda表达式、链式编程、函数式接口、Stream流式计算。
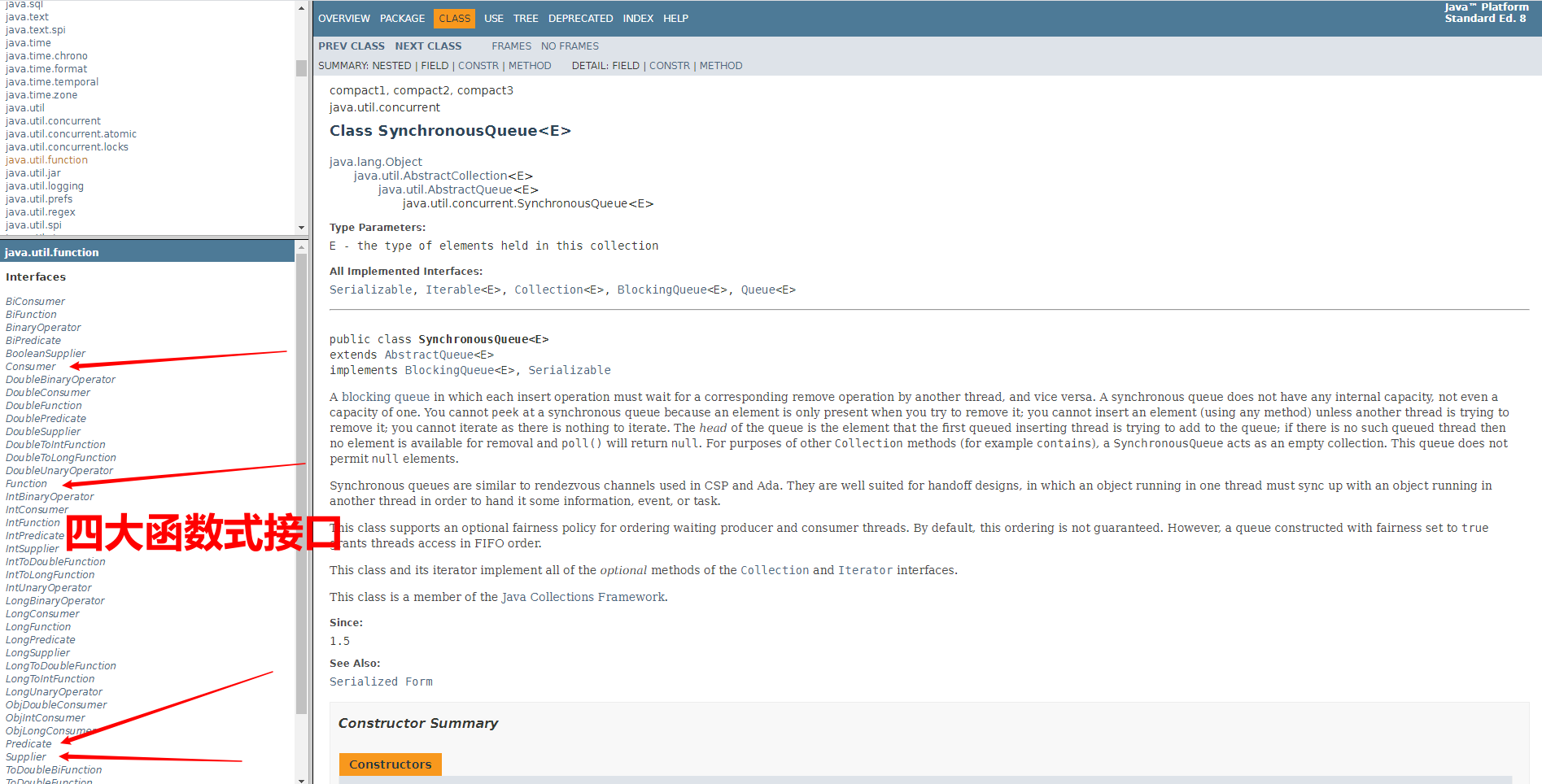
函数式接口。只有一个方法的接口。简化编程模型,在新版本的框架底层大量应用。
@FunctionalInterface
public interface Runnable {
/**
* When an object implementing interface <code>Runnable</code> is used
* to create a thread, starting the thread causes the object's
* <code>run</code> method to be called in that separately executing
* thread.
* <p>
* The general contract of the method <code>run</code> is that it may
* take any action whatsoever.
*
* @see java.lang.Thread#run()
*/
public abstract void run();
}
Function函数型接口
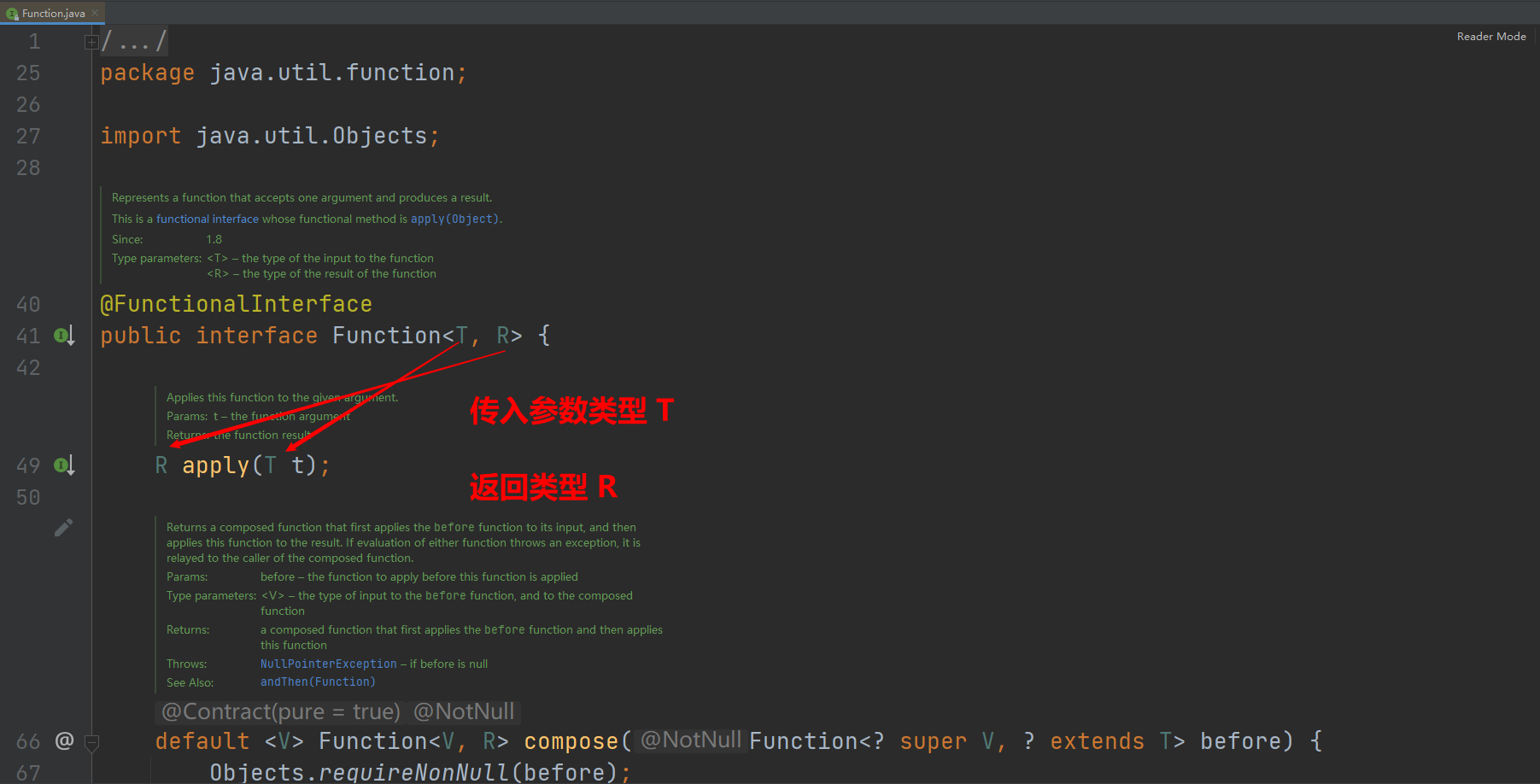
public class Demo01 {
public static void main(String[] args) {
Function function = new Function<String, String>() {
@Override
public String apply(String str) {
return str;
}
};
System.out.println(function.apply("123"));
}
}
123
Process finished with exit code 0
- 有一个输入参数,有一个输出
- 可以用lambda表达式简化
public class Demo01 {
public static void main(String[] args) {
Function function = (str) -> {
return str;
};
System.out.println(function.apply("123"));
}
}
Predicate断定型接口
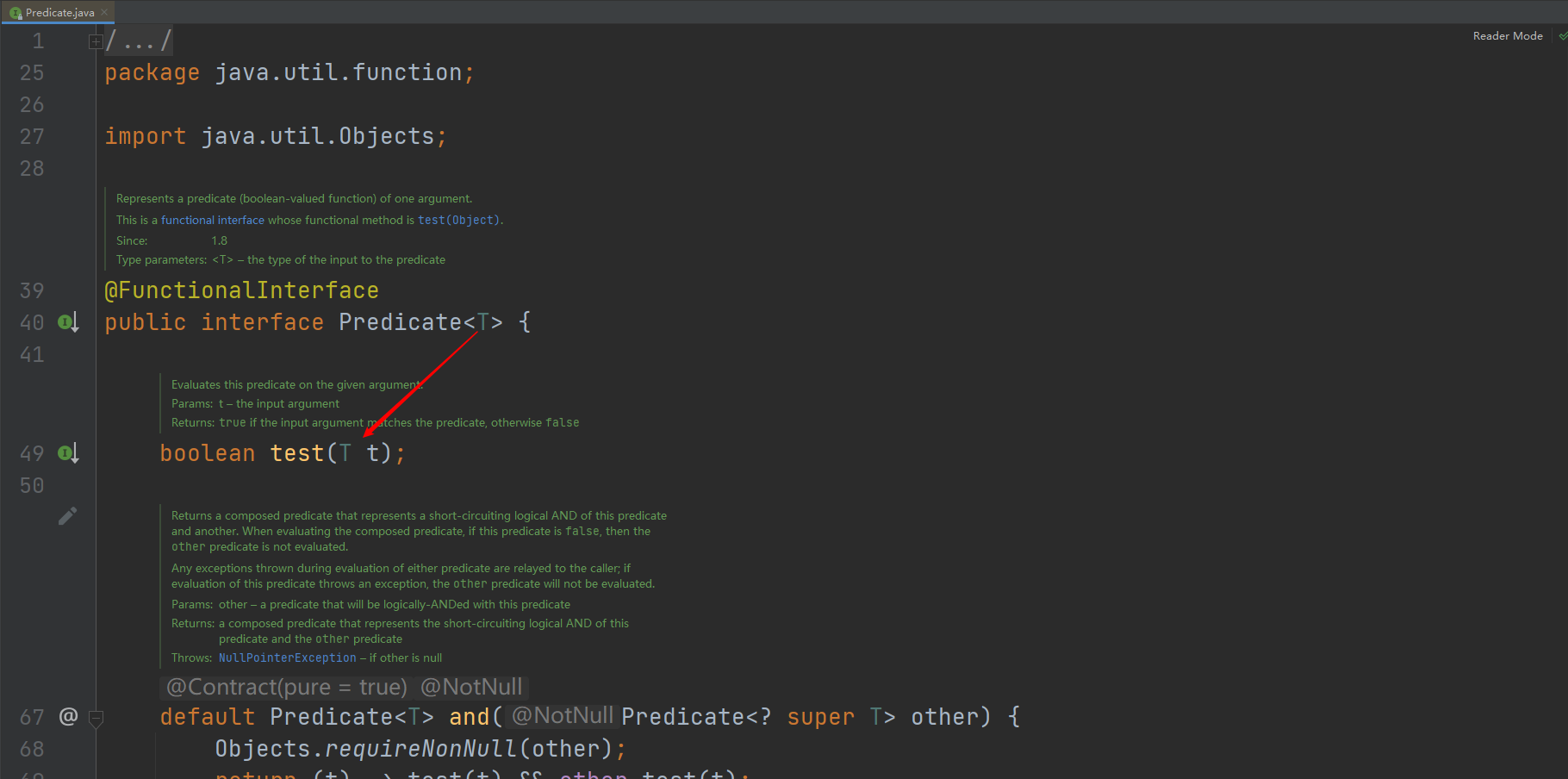
public class Demo02 {
public static void main(String[] args) {
/**
* 判断字符串是否为空
*/
Predicate predicate = new Predicate<String>() {
@Override
public boolean test(String str) {
return str.isEmpty();
}
};
System.out.println(predicate.test("123"));
}
}
false
Process finished with exit code 0
断定型接口:有一个输入参数,返回值只能是布尔值。
可以用lambda表达式简化。
public class Demo02 {
public static void main(String[] args) {
/**
* 判断字符串是否为空
*/
Predicate<String> predicate = (str) -> {
return str.isEmpty();
};
System.out.println(predicate.test("123"));
}
}
Consumer消费型接口
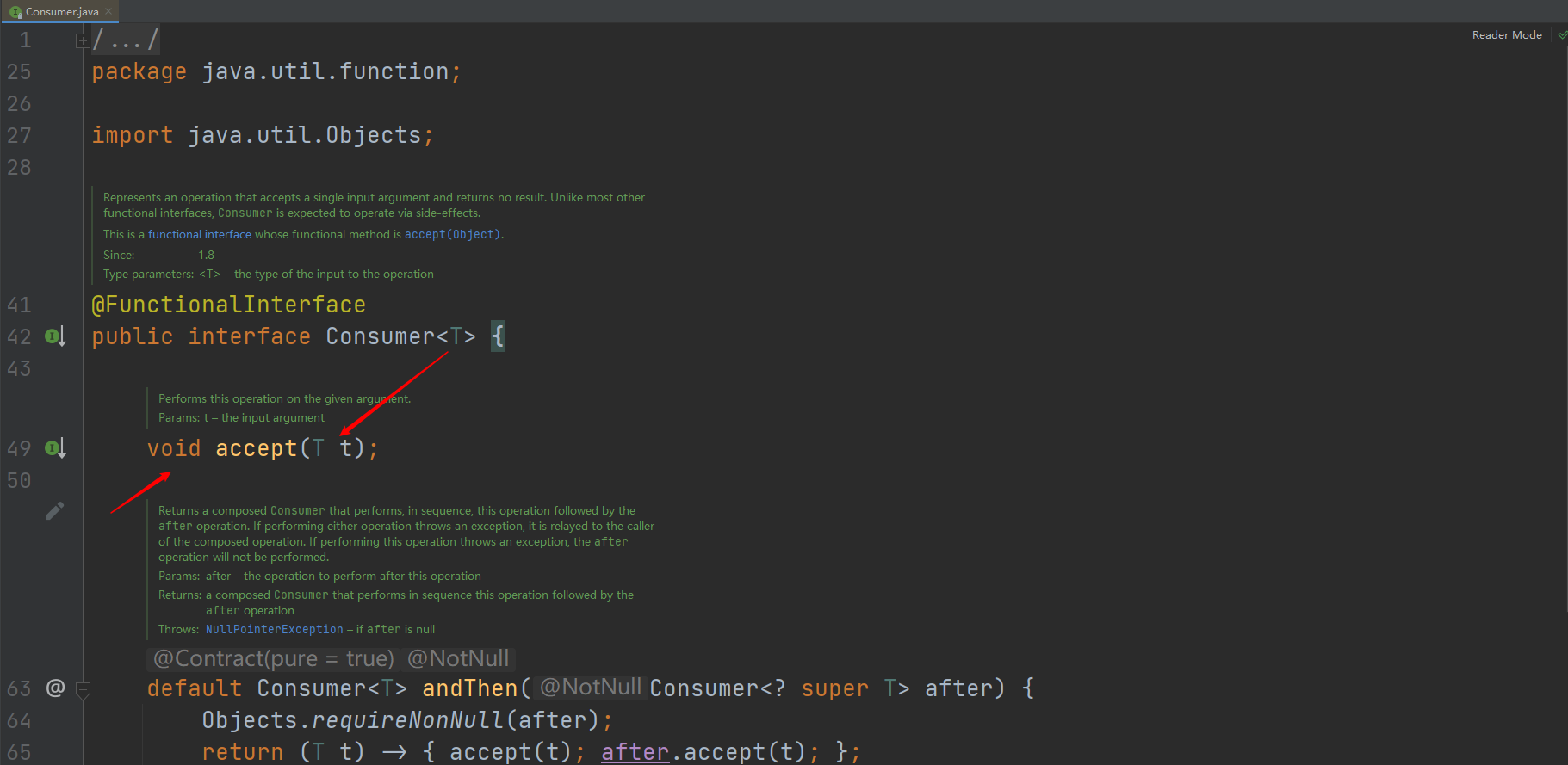
public class Demo03 {
public static void main(String[] args) {
Consumer consumer = new Consumer<String>() {
@Override
public void accept(String str) {
System.out.println(str);
}
};
consumer.accept("123");
}
}
123
Process finished with exit code 0
消费型接口:只有输入,没有返回值。
可以用lambda表达式简化。
public class Demo03 {
public static void main(String[] args) {
Consumer consumer = (str) -> {
System.out.println(str);
};
consumer.accept("123");
}
}
Supplier供给型接口
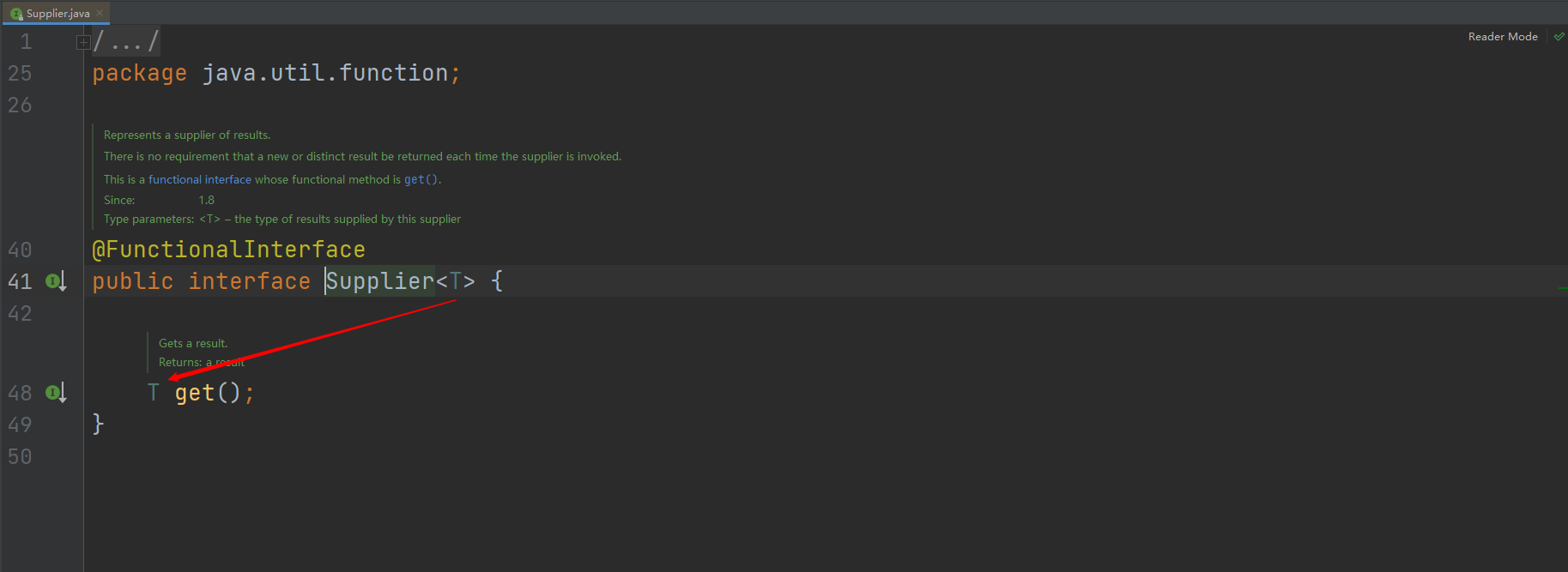
public class Demo04 {
public static void main(String[] args) {
Supplier supplier = new Supplier<Integer>() {
@Override
public Integer get() {
System.out.println("get()");
return 1024;
}
};
System.out.println(supplier.get());
}
}
get()
1024
Process finished with exit code 0
供给型接口:没有参数,只有返回值。
可以用lambda表达式简化。
public class Demo04 {
public static void main(String[] args) {
Supplier supplier = () -> {
System.out.println("get()");
return 1024;
};
System.out.println(supplier.get());
}
}